圖像旋轉是指圖像以某一點為中心旋轉一定的角度,形成一幅新的圖像的過程。當然這個點通常就是圖像的中心。既然是按照中心旋轉,自然會有這樣一個屬性:旋轉前和旋轉後的點離中心的位置不變。
基本介紹
- 中文名:圖像旋轉
- 外文名:image rotation
- 釋文:將圖像旋轉一定角度的幾何變換
- 原理:旋轉前後的點離中心的位置不變
- 隸屬:圖像處理
- 學科:計算機技術
概述
把原圖像像素從原點(x,y)放到目標位置點上(x',y'),這個(x,y)到(x',y')的轉換是經過旋轉計算而來的,那么這個圖像處理就是旋轉處理(幾何變換)。
原理
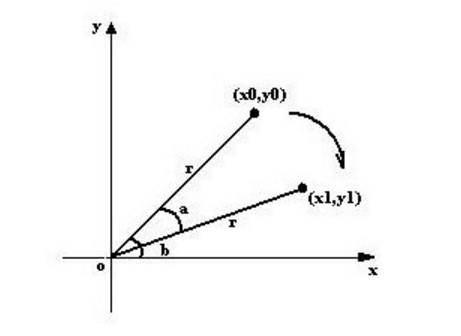
事例程式
2 #include <stdio.h>
3 #include <string>
4 #include <math.h>
5 #include <windows.h>
6 using namespace std;
7
8 #define PI 3.1415926535
9 //角度到弧度轉化
10 #define RADIAN(angle) ((angle)*PI/180.0)
11
12 void Rotation(const string& srcFile,const string& desFile,int angle)
13 {
14 BITMAPFILEHEADER bmfHeader;
15 BITMAPINFOHEADER bmiHeader;
16
17 FILE *pFile;
18 if ((pFile = fopen(srcFile.c_str(),"rb")) == NULL)
19 {
20 printf("open bmp file error.");
21 exit(-1);
22 }
23 //讀取檔案和Bitmap頭信息
24 fseek(pFile,0,SEEK_SET);
25 fread(&bmfHeader,sizeof(BITMAPFILEHEADER),1,pFile);
26 fread(&bmiHeader,sizeof(BITMAPINFOHEADER),1,pFile);
27 //先不支持小於16位的點陣圖
28 int bitCount = bmiHeader.biBitCount;
29 if (bitCount < 16)
30 {
31 exit(-1);
32 }
33 int srcW = bmiHeader.biWidth;
34 int srcH = bmiHeader.biHeight;
35 //原圖像每一行去除偏移量的位元組數
36 int lineSize = bitCount * srcW / 8;
37 //偏移量,windows系統要求每個掃描行按四位元組對齊
38 int alignBytes = ((bmiHeader.biWidth * bitCount + 31) & ~31) / 8L
39 - bmiHeader.biWidth * bitCount / 8L;
40 //原圖像快取
41 int srcBufSize = lineSize * srcH;
42 BYTE* srcBuf = new BYTE[srcBufSize];
43 int i,j;
44 //讀取檔案中數據
45 for (i = 0; i < srcH; i++)
46 {
47 fread(&srcBuf[lineSize * i],lineSize,1,pFile);
48 fseek(pFile,alignBytes,SEEK_CUR);
49 }
50 //以圖像中心為原點左上角,右上角,左下角和右下角的坐標,用於計算旋轉後的圖像的寬和高
51 POINT pLT,pRT,pLB,pRB;
52 pLT.x = -srcW/2;pLT.y = srcH/2;
53 pRT.x = srcW/2;pRT.y = srcH/2;
54 pLB.x = -srcW/2;pLB.y = -srcH/2;
55 pRB.x = srcW/2; pRB.y = -srcH/2;
56 //旋轉之後的坐標
57 POINT pLTN,pRTN,pLBN,pRBN;
58 double sina = sin(RADIAN(angle));
59 double cosa = cos(RADIAN(angle));
60 pLTN.x = pLT.x*cosa + pLT.y*sina;
61 pLTN.y = -pLT.x*sina + pLT.y*cosa;
62 pRTN.x = pRT.x*cosa + pRT.y*sina;
63 pRTN.y = -pRT.x*sina + pRT.y*cosa;
64 pLBN.x = pLB.x*cosa + pLB.y*sina;
65 pLBN.y = -pLB.x*sina + pLB.y*cosa;
66 pRBN.x = pRB.x*cosa + pRB.y*sina;
67 pRBN.y = -pRB.x*sina + pRB.y*cosa;
68 //旋轉後圖像寬和高
69 int desWidth = max(abs(pRBN.x - pLTN.x),abs(pRTN.x - pLBN.x));
70 int desHeight = max(abs(pRBN.y - pLTN.y),abs(pRTN.y - pLBN.y));
71 //分配旋轉後圖像的快取
72 int desBufSize = ((desWidth * bitCount + 31) / 32) * 4 * desHeight;
73 BYTE *desBuf = new BYTE[desBufSize];
74 //將所有像素都預置為白色
75 memset(desBuf,255,desBufSize);
76 //新圖像每一行位元組數,帶有偏移量
77 int desLineSize = ((desWidth * bitCount + 31) / 32) * 4;
78 //通過新圖像的坐標,計算對應的原圖像的坐標
79 for (i = 0; i < desHeight; i++)
80 {
81 for (j = 0; j < desWidth; j++)
82 {
83 //轉換到以圖像為中心的坐標系,並進行逆旋轉
84 int tX = (j - desWidth / 2)*cos(RADIAN(360 - angle)) + (-i + desHeight / 2)*sin(RADIAN(360 - angle));
85 int tY = -(j - desWidth / 2)*sin(RADIAN(360 - angle)) + (-i + desHeight / 2)*cos(RADIAN(360 - angle));
86 //如果這個坐標不在原圖像內,則不賦值
87 if (tX > srcW / 2 || tX < -srcW / 2 || tY > srcH / 2 || tY < -srcH / 2)
88 {
89 continue;
90 }
91 //再轉換到原坐標系下
92 int tXN = tX + srcW / 2; int tYN = abs(tY - srcH / 2);
93 //值拷貝
94 memcpy(&desBuf[i * desLineSize + j * bitCount / 8],&srcBuf[tYN * lineSize + tXN * bitCount / 8],3);
95 }
96 }
97
98 //創建目標檔案
99 HFILE hfile = _lcreat(desFile.c_str(),0);
100 //檔案頭信息
101 BITMAPFILEHEADER nbmfHeader;
102 nbmfHeader.bfType = 0x4D42;
103 nbmfHeader.bfSize = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER)
104 + desWidth * desHeight * bitCount / 8;
105 nbmfHeader.bfReserved1 = 0;
106 nbmfHeader.bfReserved2 = 0;
107 nbmfHeader.bfOffBits = sizeof(BITMAPFILEHEADER) + sizeof(BITMAPINFOHEADER);
108 //Bitmap頭信息
109 BITMAPINFOHEADER bmi;
110 bmi.biSize=sizeof(BITMAPINFOHEADER);
111 bmi.biWidth=desWidth;
112 bmi.biHeight=desHeight;
113 bmi.biPlanes=1;
114 bmi.biBitCount=bitCount;
115 bmi.biCompression=BI_RGB;
116 bmi.biSizeImage=0;
117 bmi.biXPelsPerMeter=0;
118 bmi.biYPelsPerMeter=0;
119 bmi.biClrUsed=0;
120 bmi.biClrImportant=0;
121
122 //寫入檔案頭信息
123 _lwrite(hfile,(LPCSTR)&nbmfHeader,sizeof(BITMAPFILEHEADER));
124 //寫入Bitmap頭信息
125 _lwrite(hfile,(LPCSTR)&bmi,sizeof(BITMAPINFOHEADER));
126 //寫入圖像數據
127 _lwrite(hfile,(LPCSTR)desBuf,desBufSize);
128 _lclose(hfile);
129 }
130
131 int main(int argc, char* argv[])
132 {
133 FILE *pFile;
134 if ((pFile = fopen("e://t.bmp","rb")) == NULL)
135 {
136 printf("open bmp file error.");
137 return -1;
138 }
139 string srcFile("e://t.bmp");
140 string desFile("e://Rotation.bmp");
141 Rotation(srcFile,desFile,150);
142 system("pause");
143 return 0;
144 }